I got occlusion working by creating a new material and selecting the shader VR spatial mapping occlusion, then setting the material on the AR Default Plane Mesh Renderer Component. The AR Default Plane Prefab is referenced on the AR Plane Manager Script to apply it to all detected planes. Michaelhaessig, Dec 13, 2018 #4. Occlusion Culling improves mobile game performance with OpenGL ES 3.1 and Compute Shaders by offloading work traditionally done by the CPU to the GPU. This technique significantly reduces the number of triangles that need to be drawn in scenes with massive instancing and low visibility ratios.
Unity - Ambient Occlusion artefacts. Ask Question Asked 4 years, 1 month ago. Active 4 years, 1 month ago. Viewed 1k times 0 $begingroup$ When I create two cubes from Unity Component menu. Browse other questions tagged unity shaders global-illumination ambient-occlusion. Pixel shader renders only pixels on screen, that is the area with light and shadow being visible. Pixel shader runs once on each and every pixel on the screen. The area without detailed lighting outside the camera's view is shown by Vertex Shaders.
Shader'AR Proxy' |
{ |
Properties |
{ |
} |
SubShader |
{ |
Tags |
{ |
'RenderType' = 'Transparent' |
'RenderPipeline' = 'LightweightPipeline' |
'IgnoreProjector' = 'True' |
} |
LOD300 |
Pass |
{ |
Name'AR Proxy' |
Tags |
{ |
'LightMode' = 'LightweightForward' |
} |
BlendSrcAlphaOneMinusSrcAlpha |
ZWriteOn |
CullOff |
HLSLPROGRAM |
#pragma prefer_hlslcc gles |
#pragma exclude_renderers d3d11_9x |
#pragma target 2.0 |
// ------------------------------------- |
// Material Keywords |
#pragma shader_feature _ALPHATEST_ON |
#pragma shader_feature _ALPHAPREMULTIPLY_ON |
// ------------------------------------- |
// Lightweight Pipeline keywords |
#pragma multi_compile _ _MAIN_LIGHT_SHADOWS |
#pragma multi_compile _ _MAIN_LIGHT_SHADOWS_CASCADE |
#pragma multi_compile _ _ADDITIONAL_LIGHTS_VERTEX _ADDITIONAL_LIGHTS |
#pragma multi_compile _ _ADDITIONAL_LIGHT_SHADOWS |
#pragma multi_compile _ _SHADOWS_SOFT |
// ------------------------------------- |
// Unity defined keywords |
#pragma multi_compile _ DIRLIGHTMAP_COMBINED |
#pragma multi_compile _ LIGHTMAP_ON |
//-------------------------------------- |
// GPU Instancing |
#pragma multi_compile_instancing |
#pragma vertex HiddenVertex |
#pragma fragment HiddenFragment |
#include'Packages/com.unity.render-pipelines.lightweight/ShaderLibrary/Core.hlsl' |
#include'Packages/com.unity.render-pipelines.lightweight/ShaderLibrary/Lighting.hlsl' |
struct Attributes |
{ |
UNITY_VERTEX_INPUT_INSTANCE_ID |
float4 positionOS : POSITION; |
}; |
struct Varyings |
{ |
float4 positionCS : SV_POSITION; |
float4 shadowCoord : TEXCOORD0; |
}; |
Varyings HiddenVertex(Attributes input) |
{ |
Varyings output = (Varyings)0; |
UNITY_SETUP_INSTANCE_ID(input); |
UNITY_INITIALIZE_VERTEX_OUTPUT_STEREO(output); |
VertexPositionInputs vertexInput = GetVertexPositionInputs(input.positionOS.xyz); |
output.shadowCoord = GetShadowCoord(vertexInput); |
output.positionCS = vertexInput.positionCS; |
return output; |
} |
half4HiddenFragment(Varyings input) : SV_Target |
{ |
UNITY_SETUP_STEREO_EYE_INDEX_POST_VERTEX(input); |
half s = MainLightRealtimeShadow(input.shadowCoord); |
returnhalf4(0, 0, 0, 1 - s); |
} |
ENDHLSL |
} |
UsePass'Lightweight Render Pipeline/Lit/DepthOnly' |
} |
FallBack'Hidden/InternalErrorShader' |
} |
commented May 7, 2020
I have added this to a large cube to mask content that I want to stay below the ground until it moves up above the surface. Is this going to work? In my game view the cube is bright pink. |

commented May 8, 2020
This means the shader is not compiling correctly. It's been a long time since I made this, and I haven't updated it, so it will likely require some modifications to work in newer versions of Unity / URP. You can try to take a look at this fork that is supposedly updated for URP 7.2.1. |
Learn how to use the Depth API in your own apps.
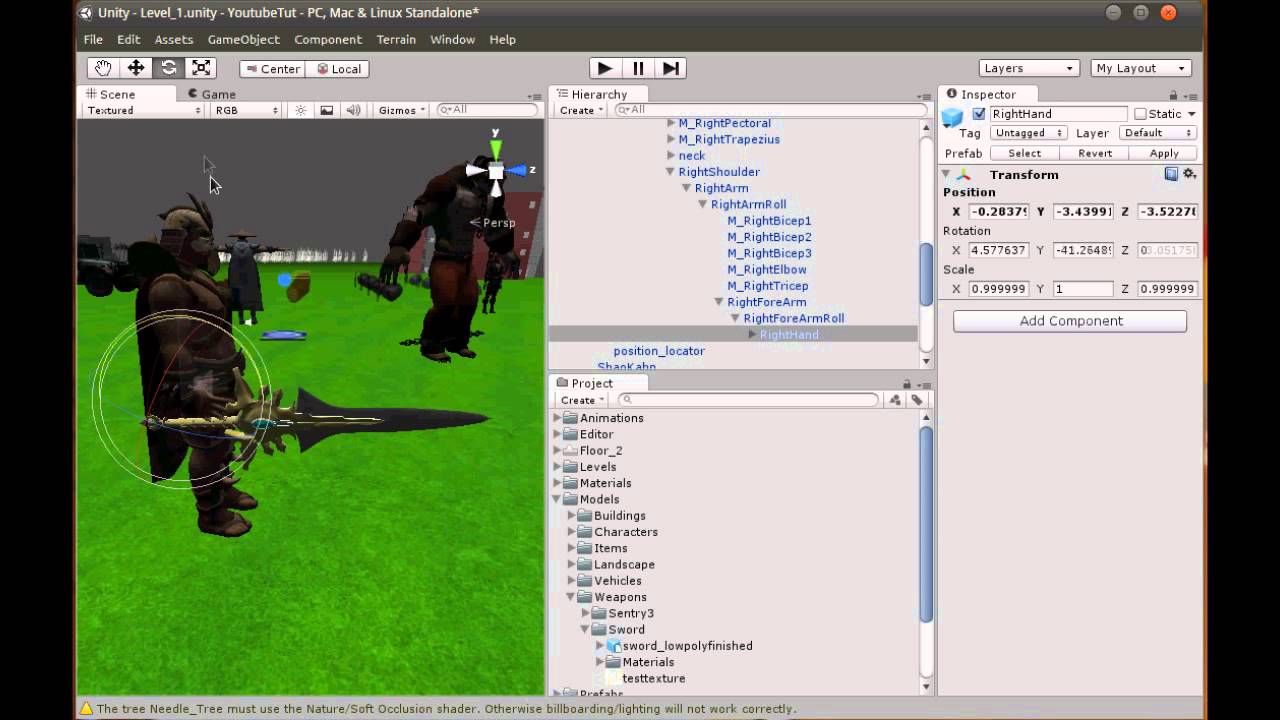
Depth API-supported devices
Only devices that are depth-supported should be able to discover depth-requiredapps in the Google Play Store. Discovery should be restricted to depth-supporteddevices when:
- A core part of the experience relies on depth
- There is no graceful fallback for the parts of the app that use depth
To restrict distribution of your app in the Google Play Store todevices that support the Depth API,add the following line to your AndroidManifest.xml
:
Check if Depth API is supported
In a new ARCore session, check whether a user's device supports the Depth API.
Retrieve depth maps
Get the depth map for the current frame.
Occlude virtual objects
Occlusion Shader Unity Patch
To occlude virtual images, include DepthEffect
as a component to the maincamera. DepthEffect
will retrieve the latest depth map and attach it,along with several properties, to the material used by this object.The OcclusionImageEffect.shader
will use the depth map to render the object with realistic occlusion.
Visualize the depth data
Unity Occlusion Probes
We provide a shader that visualizes the depth map. To see this effect, attachDepthPreview.prefab
to the main camera. DepthTexture.cs
is acomponent in the depth preview object. CameraColorRampShader.shader
will use the depth map to render a visualization, using a color ramp torepresent different distances from the camera.
Conventional versus alternative implementations of occlusion rendering
Nature Soft Occlusion Shader Unity
The HelloAR sample appuses a two-pass rendering configuration to simulate occlusion. The first(render) pass renders all of the virtual content into an intermediary buffer.The second pass uses the depth map to combine the virtual content with thereal world camera. The efficiency of each approach depends on the complexity ofthe scene and other app-specific considerations. The two-pass approach requiresno additional shader work.
An alternative way to render occlusion is to implement per-object, forward-passrendering. As each object is rendered, the app uses the depth map to determinewhether certain pixels in the virtual content are visible. If the pixels are notvisible, they will be clipped, simulating occlusion on the user’s device.
We recommend tailoring the code in OcclusionImageEffect.shader
and CameraColorRampShader.shader
to your specific application.
Alternative uses of the Depth API
The HelloAR app uses the Depth API to create depth maps and simulateocclusion. Other uses for the Depth API include:
- Collisions: virtual objects bouncing off of real world objects
- Distance measurement
- Re-lighting a scene
- Re-texturing existing objects: turning a floor into lava
- Depth-of-field effects: blurring out the background or foreground
- Environmental effects: fog, rain, and snow

Unity Occlusion Map
For more detail and best practices for applying occlusion in shaders, check outthe HelloAR sample app.
Understanding depth values
Given point A
on the observed real-world geometry and a 2D point a
representing the same point in the depth image, the value given by the DepthAPI at a
is equal to the length of CA
projected onto the principal axis.This can also be referred as the z-coordinate of A
relative to the cameraorigin C
. When working with the Depth API, it is important to understand thatthe depth values are not the length of the ray CA
itself, but the projectionof it.
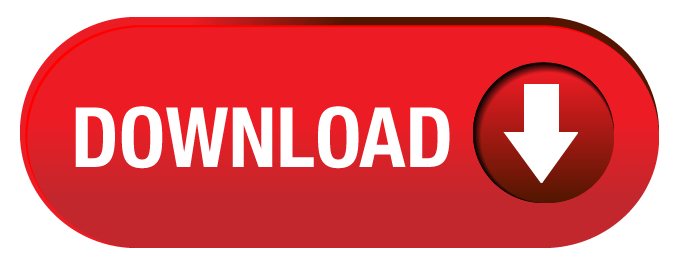